注釈
Go to the end to download the full example code.
ブール演算#
閉じた(多様な)曲面でブーリアン演算を行う.
ブール/位相演算(交差,結合,切断)法は, pyvista.PolyData
メッシュタイプに対してのみ実装され,任意の pyvista.PolyData
メッシュから直接アクセス可能である. pyvista.PolyDataFilters
をチェックして,次のフィルタを確認してください.
基本的に,ブーリアン結合,切断,交差はすべて同じ操作です.ただ,オブジェクトの異なる部分が最終的に保持されるだけです.
演算子 -
は,PyVistaの任意の2つの pyvista.PolyData
メッシュの間で使用することができ,最初のメッシュを2番目のメッシュでカットします. これらのメッシュはすべて3角形のメッシュでなければなりません.これは pyvista.PolyData.is_all_triangles
で確認できます.
注釈
マージでは, +
演算子をPyVistaの任意の2つのメッシュ間で使用できます.この演算子は,任意の2つのメッシュを結合するために .merge()
フィルタを呼び出すだけです.これは単に boolean_union
フィルタを呼び出すだけでのPyVistaの |
演算子とは異なり、結果に対して追加の計算を行うことなく2つのメッシュを重ね合わせます。 PyVista の &
演算子は単に boolean_intersection
を呼び出します。
警告
ブーリアン演算が思った通りに反応しない(例えば,間違った部分が消えてしまう)場合,メッシュの1つが法線を内側に向けている可能性があります.法線を可視化するには, pyvista.PolyDataFilters.plot_normals()
を使用してください.
from __future__ import annotations
import pyvista as pv
sphere_a = pv.Sphere()
sphere_b = pv.Sphere(center=(0.5, 0, 0))
ブール演算和#
pyvista.PolyDataFilters.boolean_union()
フィルタを使用して, A
と B
のブール結合を行います.
2つの多様体メッシュ A
と B
の結合は, A
にあるメッシュ, B
にあるメッシュ,または A
と B
の両方にあるメッシュとなります.
論理和にはオペランドの順序は関係ありません(演算は可換です).
result = sphere_a | sphere_b
pl = pv.Plotter()
_ = pl.add_mesh(sphere_a, color='r', style='wireframe', line_width=3)
_ = pl.add_mesh(sphere_b, color='b', style='wireframe', line_width=3)
_ = pl.add_mesh(result, color='lightblue')
pl.camera_position = 'xz'
pl.show()
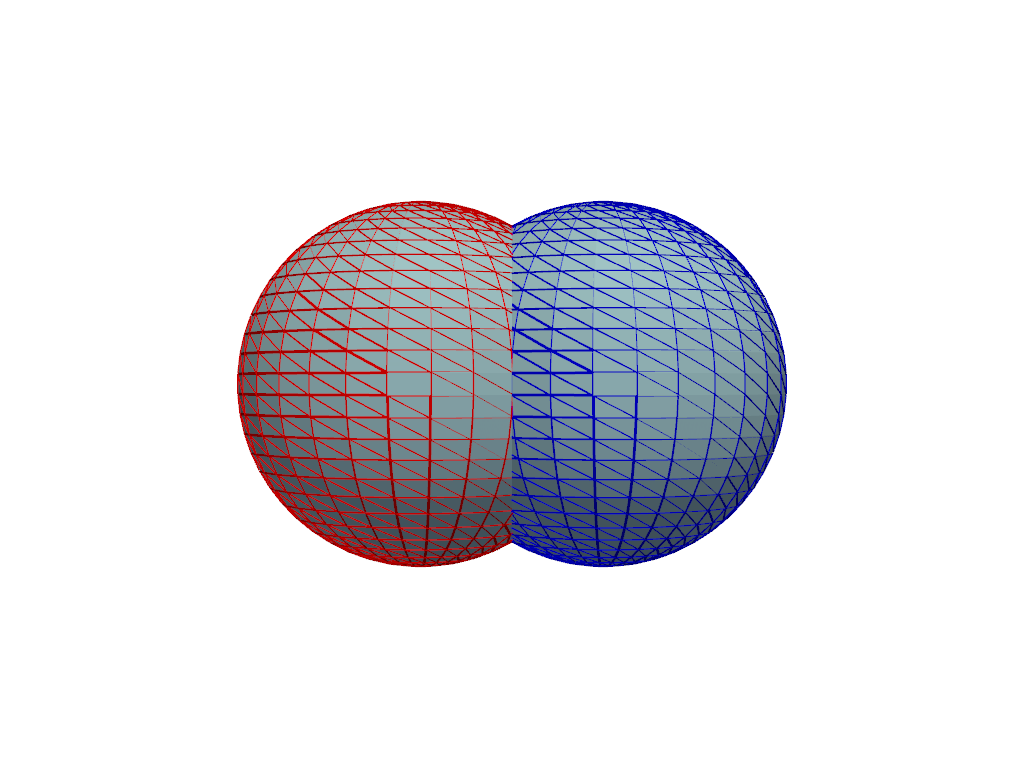
論理差#
両方のメッシュが pyvista.PolyData
であるため, pyvista.PolyDataFilters.boolean_difference()
フィルタまたは -
演算子を使用して A
と B
のブーリアン値の差を実行します.
2つのマニフォールドメッシュ A
と B
の差は, A
のメッシュのうち B
に属さない部分の体積である.
真偽判定にはオペランドの順序が重要です.
result = sphere_a - sphere_b
pl = pv.Plotter()
_ = pl.add_mesh(sphere_a, color='r', style='wireframe', line_width=3)
_ = pl.add_mesh(sphere_b, color='b', style='wireframe', line_width=3)
_ = pl.add_mesh(result, color='lightblue')
pl.camera_position = 'xz'
pl.show()
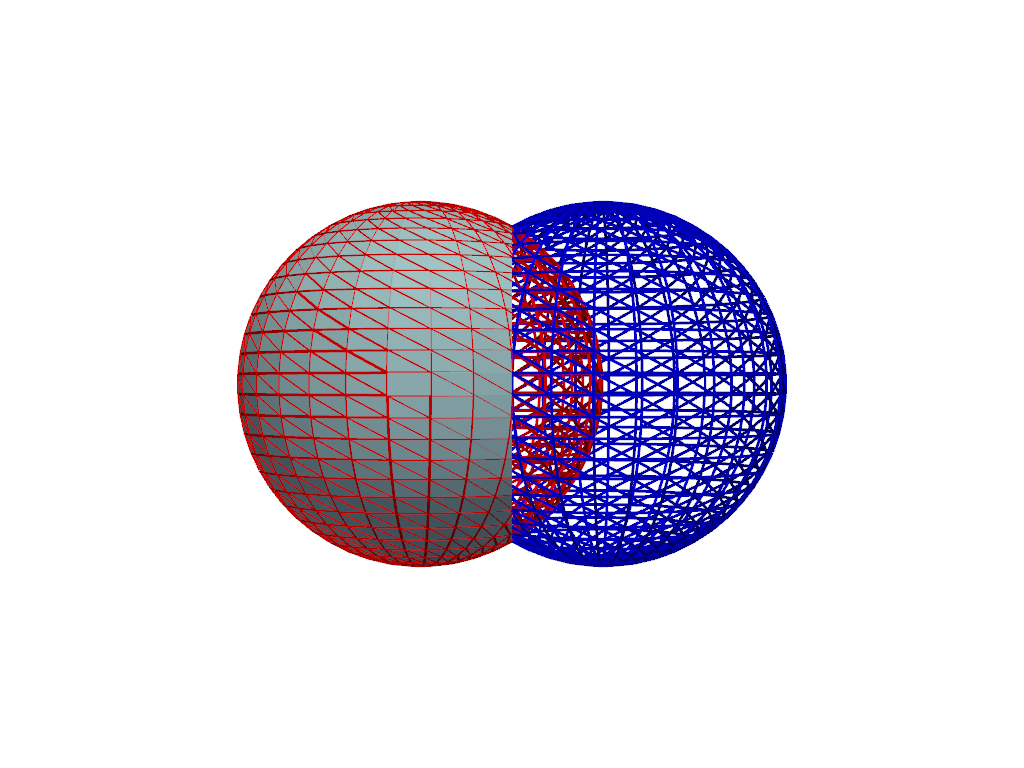
ブール交差#
pyvista.PolyDataFilters.boolean_intersection()
フィルタを使用して, A
と B
のブール交差を行います.
2つの多様体メッシュ A
と B
の交点は, A
の体積が B
の中にもあるメッシュである.
論理交差にはオペランドの順序は関係ありません(演算は可換です).
result = sphere_a & sphere_b
pl = pv.Plotter()
_ = pl.add_mesh(sphere_a, color='r', style='wireframe', line_width=3)
_ = pl.add_mesh(sphere_b, color='b', style='wireframe', line_width=3)
_ = pl.add_mesh(result, color='lightblue')
pl.camera_position = 'xz'
pl.show()
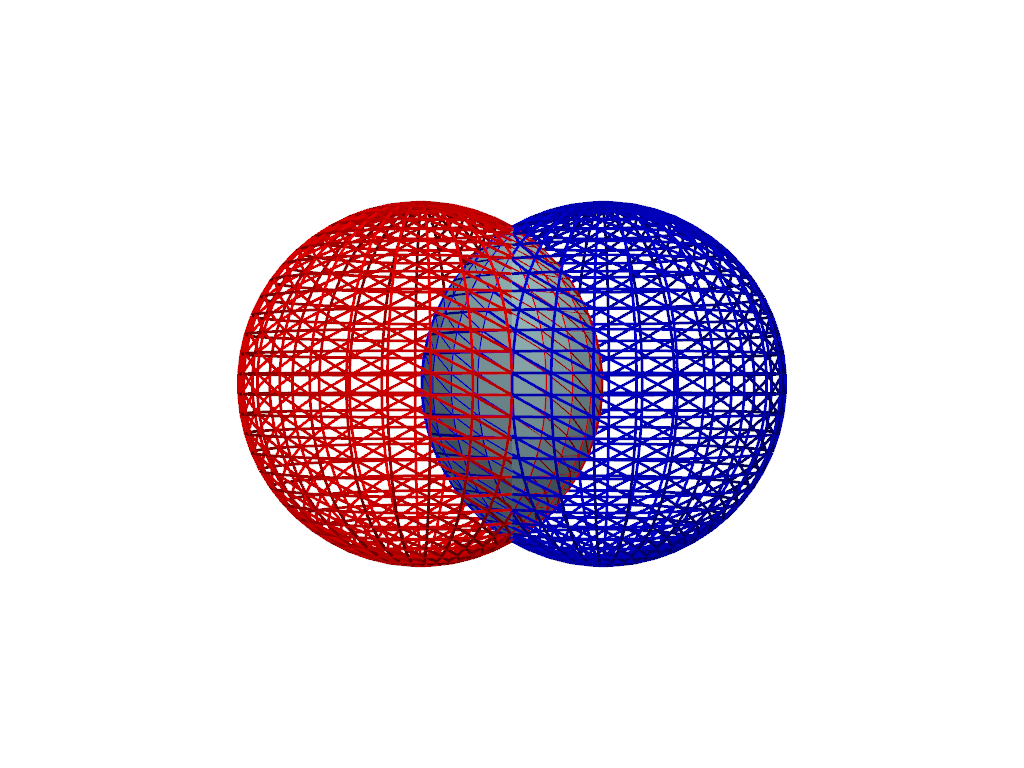
Behavior due to flipped faces#
Note that these boolean filters behave differently depending on the
orientation of the faces. This is because the orientation determines
which parts are considered to be the "outside" or the "inside" portion
of the mesh. This example uses flip_faces()
to flip the faces.
Boolean difference with both cube and sphere faces oriented outward. This is the "normal" behavior.
cube = pv.Cube().triangulate().subdivide(3)
sphere = pv.Sphere(radius=0.6)
result = cube.boolean_difference(sphere)
result.plot(color='lightblue')
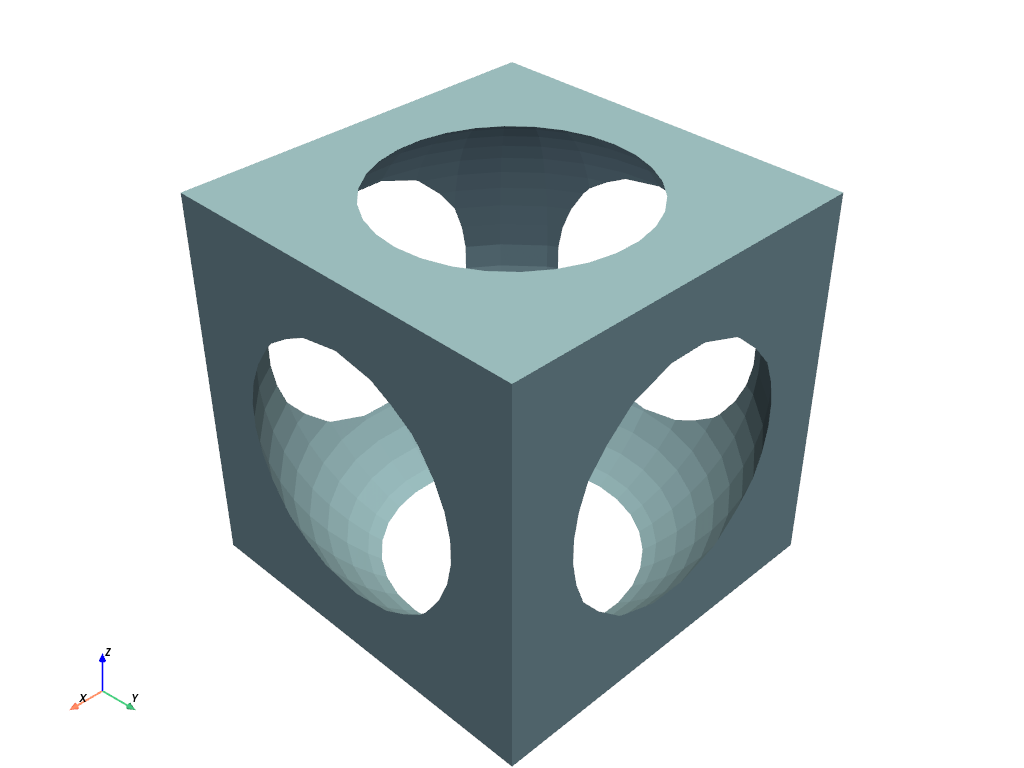
Boolean difference with cube faces outward, sphere faces inward.
cube = pv.Cube().triangulate().subdivide(3)
sphere = pv.Sphere(radius=0.6).flip_faces()
result = cube.boolean_difference(sphere)
result.plot(color='lightblue')
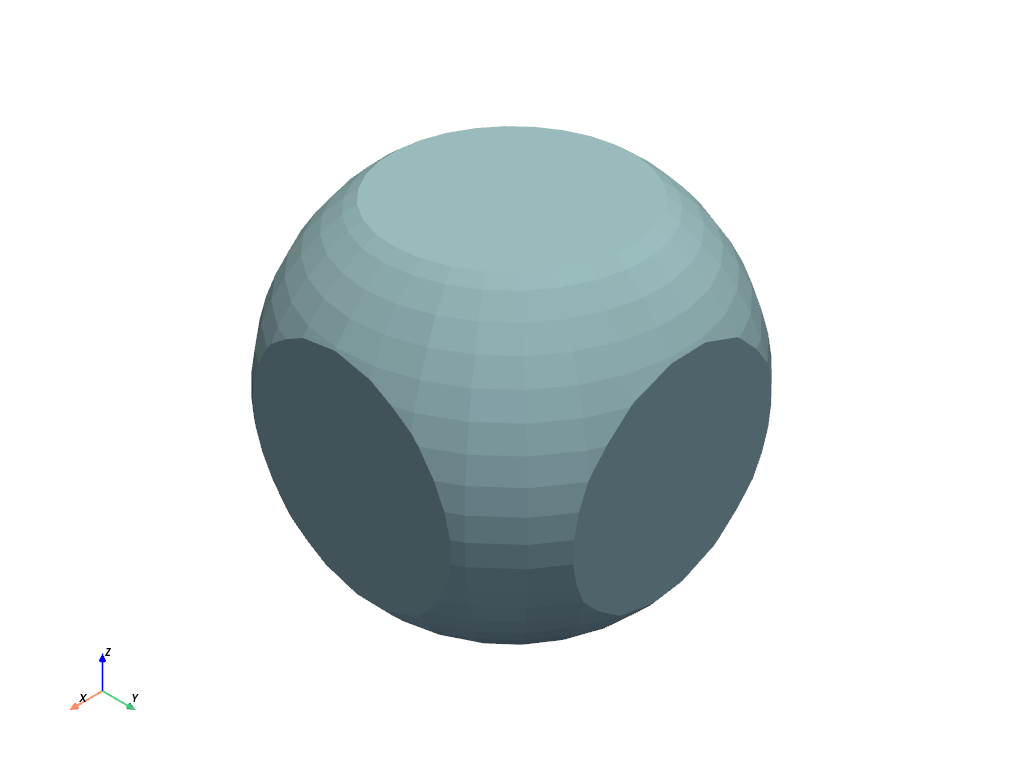
Boolean difference with cube faces inward, sphere faces outward.
cube = pv.Cube().triangulate().subdivide(3).flip_faces()
sphere = pv.Sphere(radius=0.6)
result = cube.boolean_difference(sphere)
result.plot(color='lightblue')
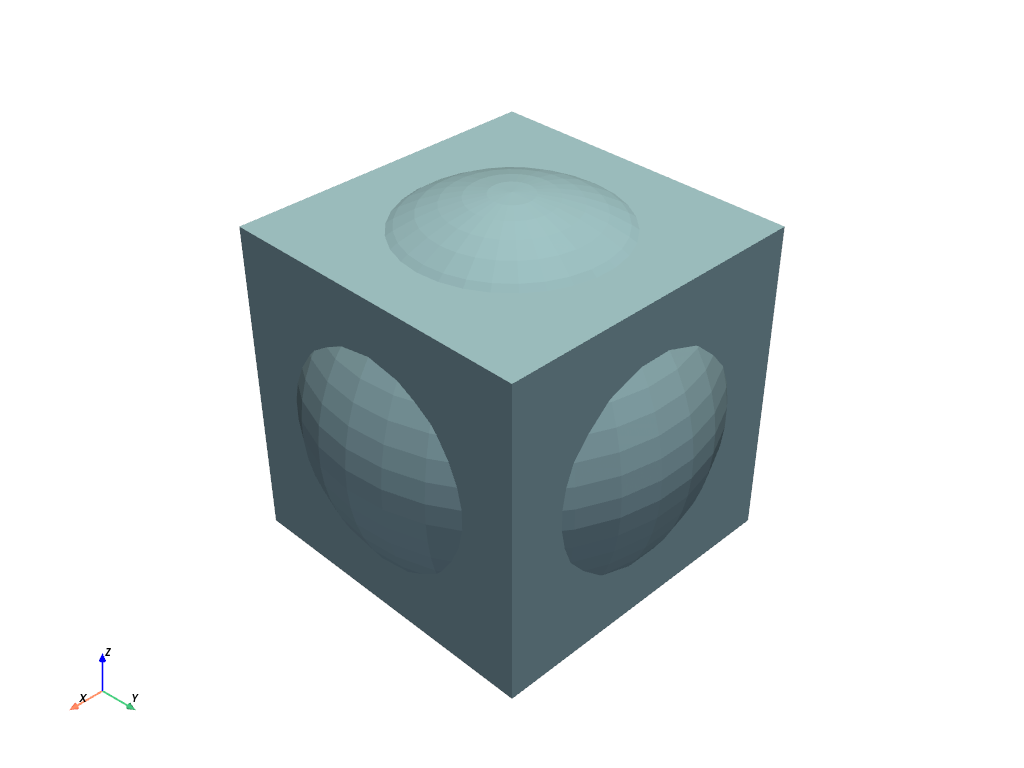
Both cube and sphere faces inward.
cube = pv.Cube().triangulate().subdivide(3).flip_faces()
sphere = pv.Sphere(radius=0.6).flip_faces()
result = cube.boolean_difference(sphere)
result.plot(color='lightblue')
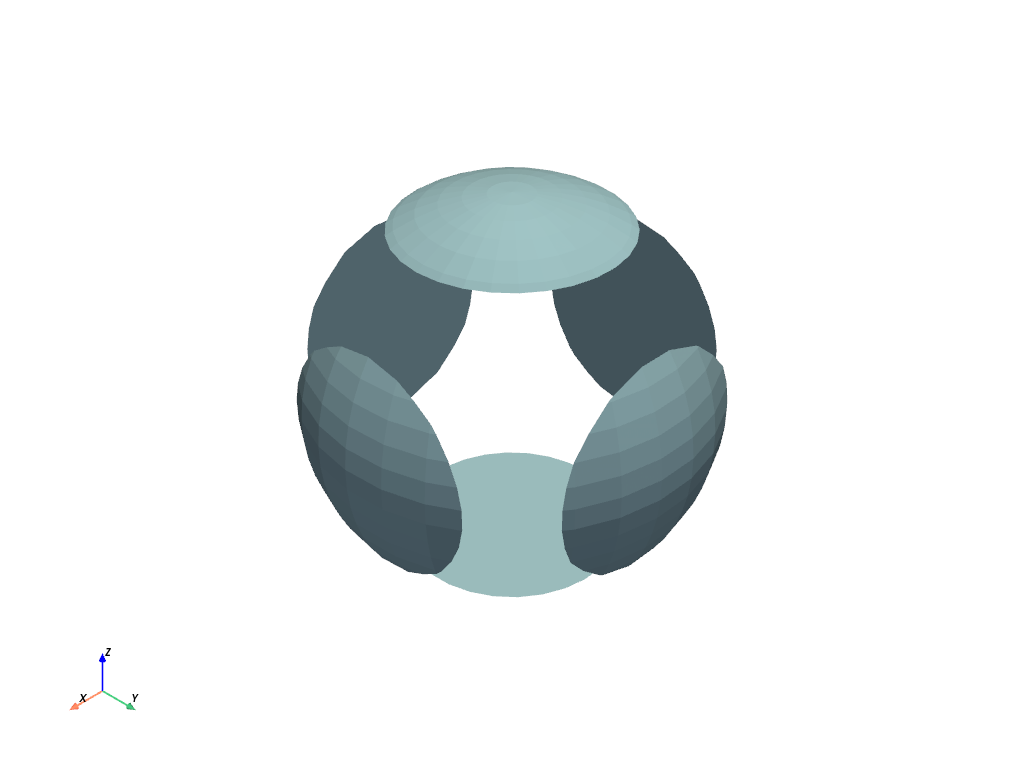
Total running time of the script: (0 minutes 6.109 seconds)