注釈
Go to the end to download the full example code
3角形サーフェスを作成#
一連のポイントからDelaunay3角形分割を介してサーフェスを作成します.
import numpy as np
import pyvista as pv
単純な軌道#
まず,表面のポイントをいくつか作成します.
# Define a simple Gaussian surface
n = 20
x = np.linspace(-200, 200, num=n) + np.random.uniform(-5, 5, size=n)
y = np.linspace(-200, 200, num=n) + np.random.uniform(-5, 5, size=n)
xx, yy = np.meshgrid(x, y)
A, b = 100, 100
zz = A * np.exp(-0.5 * ((xx / b) ** 2.0 + (yy / b) ** 2.0))
# Get the points as a 2D NumPy array (N by 3)
points = np.c_[xx.reshape(-1), yy.reshape(-1), zz.reshape(-1)]
points[0:5, :]
array([[-201.19542481, -204.62576987, 1.62841142],
[-182.99500028, -204.62576987, 2.30995119],
[-159.15511525, -204.62576987, 3.47316876],
[-137.22964657, -204.62576987, 4.80658141],
[-113.1538541 , -204.62576987, 6.49735497]])
これらのポイントを使用して,ポイントクラウドPyVistaデータオブジェクトを作成します.これは pyvista.PolyData
オブジェクトに含まれます.
# simply pass the numpy points to the PolyData constructor
cloud = pv.PolyData(points)
cloud.plot(point_size=15)
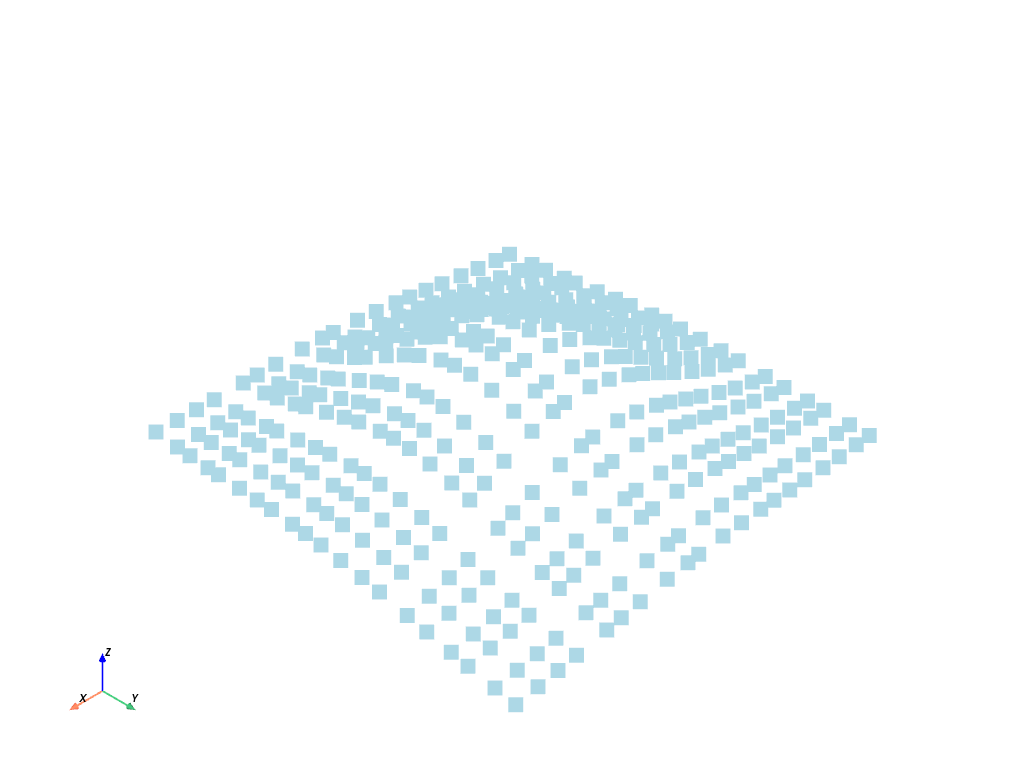
ポイントのPyVistaデータ構造ができたので,3角形分割を実行して,これらの退屈な個別のポイントを接続されたサーフェスに変えることができます.
surf = cloud.delaunay_2d()
surf.plot(show_edges=True)
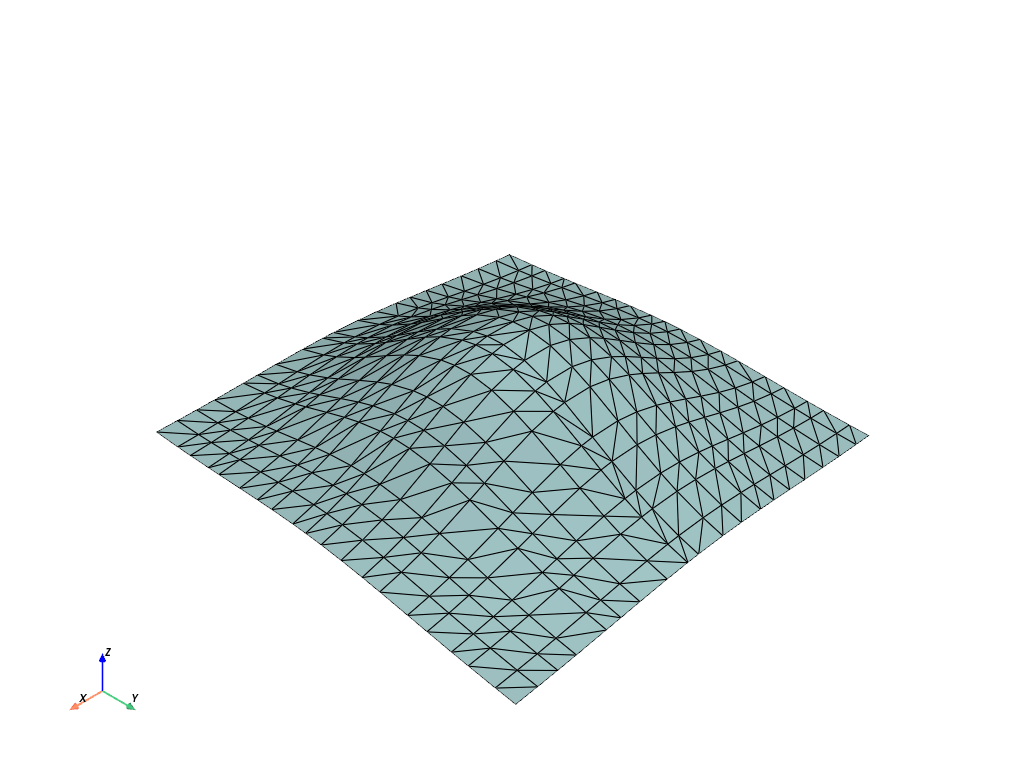
マスクされた3角形#
x = np.arange(10, dtype=float)
xx, yy, zz = np.meshgrid(x, x, [0])
points = np.column_stack((xx.ravel(order="F"), yy.ravel(order="F"), zz.ravel(order="F")))
# Perturb the points
points[:, 0] += np.random.rand(len(points)) * 0.3
points[:, 1] += np.random.rand(len(points)) * 0.3
# Create the point cloud mesh to triangulate from the coordinates
cloud = pv.PolyData(points)
cloud
これらの点で3角形分割を実行します
surf = cloud.delaunay_2d()
surf.plot(cpos="xy", show_edges=True)
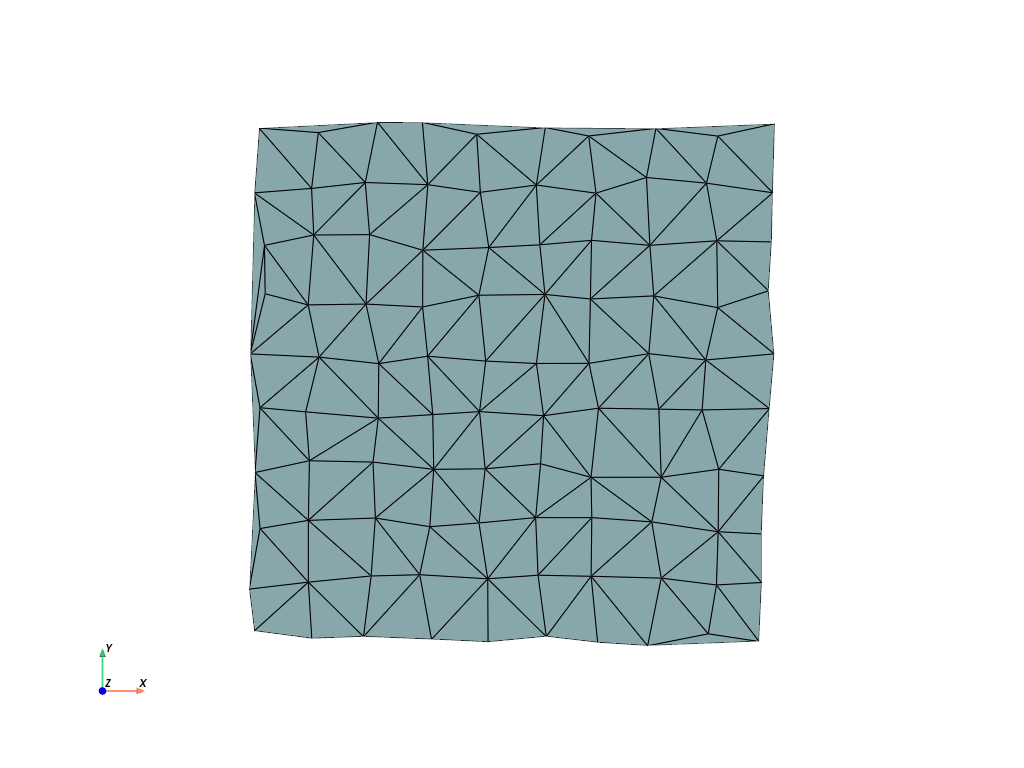
外側のエッジの一部は拘束されておらず,3角形分割によって不要な3角形が追加されていることに注意してください.私たちは alpha
パラメータでそれを緩和します.
surf = cloud.delaunay_2d(alpha=1.0)
surf.plot(cpos="xy", show_edges=True)
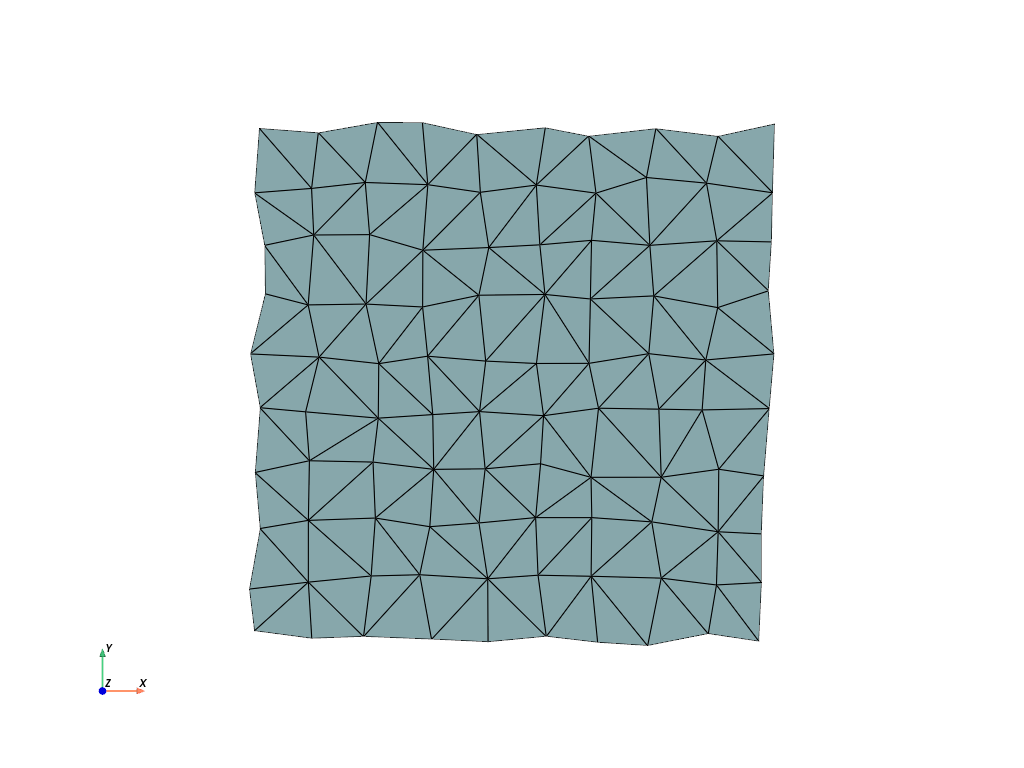
また, edge_source
パラメータを使用して,3角形分割中に無視するポリゴンを追加することもできます.
# Define a polygonal hole with a clockwise polygon
ids = [22, 23, 24, 25, 35, 45, 44, 43, 42, 32]
# Create a polydata to store the boundary
polygon = pv.PolyData()
# Make sure it has the same points as the mesh being triangulated
polygon.points = points
# But only has faces in regions to ignore
polygon.faces = np.insert(ids, 0, len(ids))
surf = cloud.delaunay_2d(alpha=1.0, edge_source=polygon)
p = pv.Plotter()
p.add_mesh(surf, show_edges=True)
p.add_mesh(polygon, color="red", opacity=0.5)
p.show(cpos="xy")
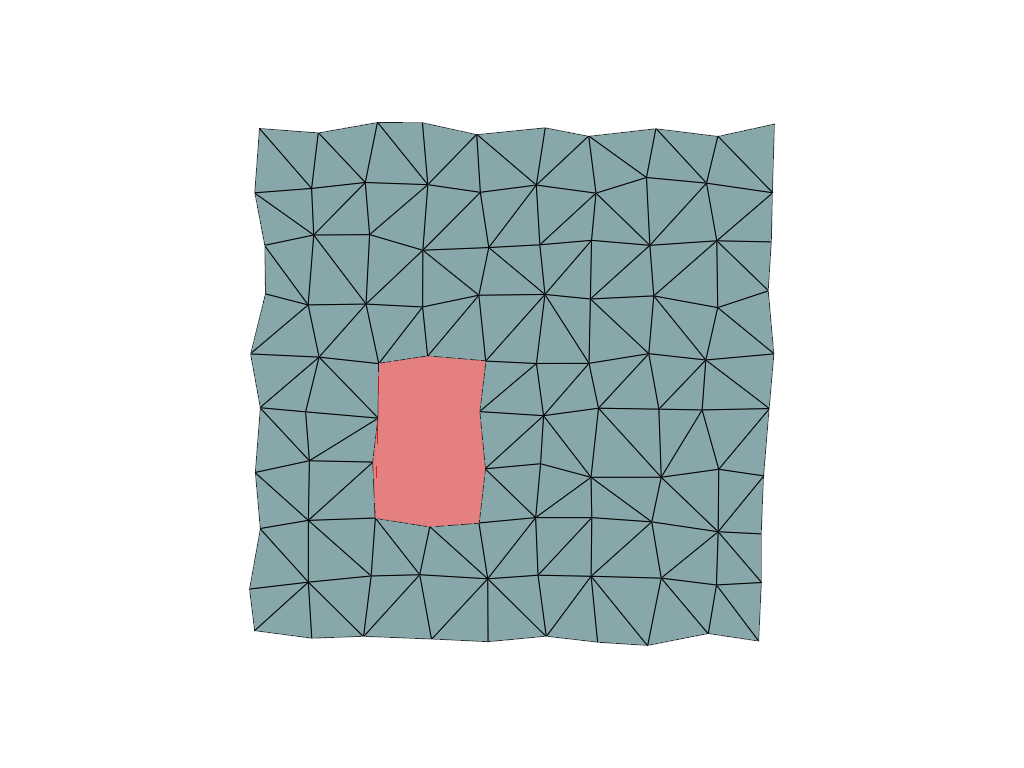
Total running time of the script: (0 minutes 1.190 seconds)